As autonomous software development continues to evolve, the way we turn ideas into software has changed significantly. In the early days, people had to set switches by hand. Now, we have smart tools powered by AI that help design software. Each step shows how humans want to connect their thinking with how machines work. This article looks at that journey and shows how the programmer’s role has changed, leading to AI becoming the ‘human compiler’—a crucial step toward AI moving towards autonomous software development.
The Layers of Abstraction in Programming Evolution
To understand how programming has evolved, it’s helpful to see it as a series of abstraction layers. First, each layer automates tasks that programmers once had to do by hand. As a result, this raises the level of abstraction, allowing them to focus on bigger ideas. Over time, with each new generation of tools, one layer of manual work is removed from the bottom up. Consequently, programmers begin to trust these tools to manage the smaller, lower-level details..
Flipping Switches (Machine Code)
At this most basic level, the programmer had to mentally go through all the layers, from the highest level of abstraction down to the physical manipulation of the machine:
Business Problem → Logical Solution → Procedures & Data Manipulation → Machine Instructions → Physical Implementation.
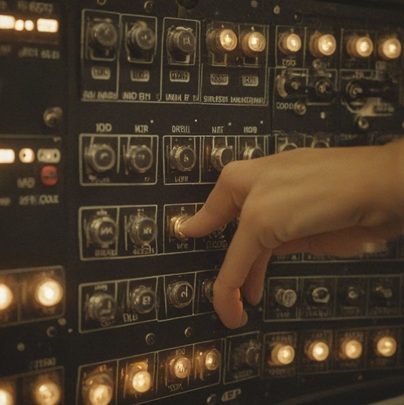
This era demanded a deep understanding of both the business problem and the inner workings of the machine. Programming meant interacting directly with the hardware by physically manipulating switches, wires, and punch cards. This approach was incredibly tedious and error-prone, requiring meticulous attention to detail and an intimate understanding of the underlying hardware.
Example:
10111000 00000001 11000000 00000000
This snippet represents a simple machine instruction to move a byte into a register—every bit must be precise.
Talking to the Machine (Assembly Language)
AAssembly language removed the need for physical implementation, which was the first layer. Before this, programmers had to manually control the machine’s hardware. However, with assembly language, they could use mnemonics to represent instructions. These were then translated into machine code by an assembler. Although this still required a solid understanding of the machine’s architecture, it was a big improvement over flipping switches.
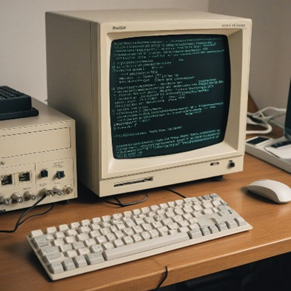
Example (Assembly Language):
MOV AL, 1
MOV BL, 2
ADD AL, BL
This code moves values into registers and adds them together.
Writing Recipes (C, C++)
High-level languages like C and C++ took the abstraction even further by removing the need to think in terms of machine instructions, which was the second layer. Instead of focusing on low-level details, developers could write code that was easier to read and understand. At the same time, these languages still gave them control over system resources. While programmers no longer worked directly with registers, they still had to manage memory allocation and write algorithms manually.
Example (C Language):
#include <stdio.h>
int main() {
int a = 1;
int b = 2;
int sum = a + b;
printf("Sum: %d\n", sum);
return 0;
}
Here, the code is more abstract and easier to read.
Solving Problems (SQL, Java, Python, C#, Perl, PHP, Report Generators)
As programming languages continued to evolve, the focus gradually shifted from writing detailed instructions to solving specific problems in different fields. This change led to the creation of specialized languages and tools designed to handle particular tasks more efficiently.
For example, these languages and tools provided higher-level abstractions and features tailored to certain jobs. SQL makes it easy to interact with databases, while report generators help simplify the creation of complex reports. Similarly, languages like Perl and PHP offer powerful tools for web development and scripting. Meanwhile, Java and C# provide strong frameworks for building complex applications, including features like garbage collection for automatic memory management.
Example (Java):
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public static void main(String args) {
Calculator calc = new Calculator();
System.out.println("Sum: " + calc.add(1, 2));
}
}
In this Java example, the complexities of memory management are hidden, and the focus shifts to organizing logic within objects to represent and manipulate business concepts.
AI as Human Compiler: The Path to Trust and System-Level Design
AI-driven development marks a major shift, with the potential for AI to act as the “human compiler.” However, we’re not quite there yet. While AI shows impressive skills in generating code—turning simple natural language requests into working code snippets—it still doesn’t fully meet the “human compiler” ideal.
For instance, imagine a product manager describing a new feature in plain language. In this scenario, an AI system would not only generate the code but also integrate it into the existing codebase, run tests, and deploy it to production. As a result, the developer’s role would shift to setting goals, aligning with business objectives, and solving complex problems that require human intuition and creativity.
In short, AI will become the human compiler, turning human ideas directly into working software. This will make software development more accessible, allowing people with little or no coding experience to build advanced applications. However, developers will need new skills, such as guiding AI, ensuring ethical standards, and overseeing automated processes.
The future of software development is exciting, where human creativity and AI power come together to create a new wave of innovation and efficiency.
That said, it’s important to understand that this journey will happen in stages:
AI as the Coder’s Assistant: Enhancing Development Efficiency:
Initially, AI will serve as a powerful assistant, helping developers write, debug, and optimize code more efficiently. At this stage, AI tools will support coders by automating routine tasks and offering suggestions based on clear instructions from their development leads. For example, coders, who usually focus on turning detailed specifications into functional code, will benefit from AI’s ability to quickly generate code snippets, spot potential errors, and recommend optimizations.
This collaboration between coders and AI will make the development process smoother, cut down on repetitive tasks, and improve both the speed and accuracy of code production. As a result, coders will be able to deliver high-quality software faster, while still using their expertise to tackle the creative and complex parts of development
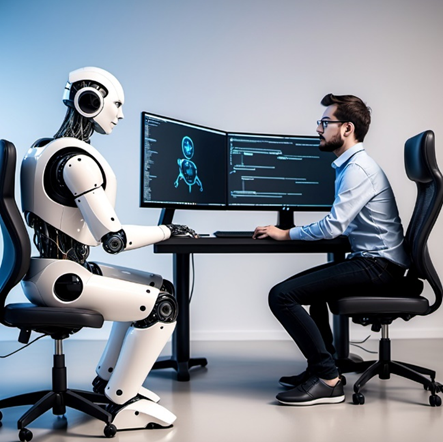
Example (AI-Generated Code):
Prompt:
Create a function to add two numbers and print the result.
AI Output:
def add_numbers(a, b):
sum = a + b
print(f"Sum: {sum}")
add_numbers(1, 2)
his example, while impressive, also shows the limits of current AI code generation. While AI can handle simple prompts well, it struggles with the complexity and subtle details of larger software systems. For instance, imagine trying to build a full e-commerce platform using only natural language prompts! The detailed work involved in user authentication, product catalogs, shopping carts, and payment processing would quickly overwhelm this approach.
Read more about AI-Driven Development: The AI-Driven Development Glossary.
Farewell Coders: Lead Developers Steering AI Teams:
n the next phase, lead developers will oversee multiple AI code generation tools, making sure the code produced is accurate, consistent, and follows architectural standards. As AI continues to improve and handles more complex coding tasks, the role of traditional coders will slowly decrease. Instead, the focus will shift to tech leads who have a deep understanding of both the technical details and the strategic goals of software development.
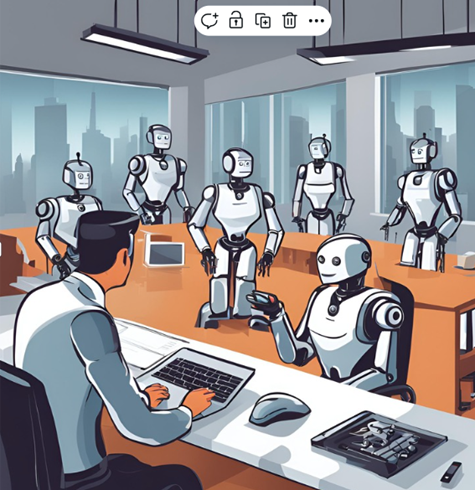
These tech leads will be responsible for overseeing the AI tools and ensuring they produce high-quality code that meets the project’s requirements. Additionally, they will play a key role in guiding the AI, providing high-level direction, and making strategic decisions about the software’s architecture and design. To successfully manage this shift, tech leads will need to develop new skills, such as understanding AI algorithms, managing automated processes, and ensuring that ethical considerations are addressed.
As AI takes over routine coding tasks, tech leads will shift their focus to more complex problem-solving, innovation, and maintaining overall oversight of the development process. This evolution will create a more efficient and innovative software development environment, where human creativity and AI capabilities collaborate to reach new levels of productivity and quality.
The Human Compiler: Codifying Human Intent:
In the final stage, AI will take on the majority of coding tasks, effectively replacing developers in routine coding processes. Humans will shift to providing high-level direction, focusing on architecture, design, and strategic oversight, while AI handles the implementation.
The way to the Human compiler
To truly become the “human compiler,” AI needs to evolve in two key areas:
Building Trust and Reliability
AI must generate code that is so reliable and predictable that programmers no longer need to interact with it. This will require advancements in AI’s understanding of intent, its ability to produce robust and maintainable code, and potentially, greater transparency in its reasoning. The ultimate goal is for the AI’s “compilation” process to become a trusted black box—reliable, just like a traditional compiler.
Scaling to System-Level Design
AI must expand beyond generating small code snippets and develop the ability to handle system-level design. It should be capable of taking high-level architectural descriptions and converting them into functional code, managing complexities such as dependencies, integrations, and overall system design. This shift will move AI from creating code snippets to generating entire software systems.
However, simply increasing the complexity of prompts, as seen in the example below, is not a sustainable solution.
Example (Hypothetical AI System Design Language – Illustrative, Not Scalable):
System: User Management Service
Components:
- REST API: Handles HTTP requests.
- User Database: Stores user information.
- Authentication Module: Manages login and security.
Endpoints:
- POST /users: Create a new user.
- PUT /users/{id}: Update an existing user.
- DELETE /users/{id}: Delete a user.
Database Schema:
- Table: Users
- id: Integer (Primary Key)
- name: String
- email: String
- password: String
Business Logic:
1. Validate input data.
2. Hash passwords before storing.
3. Ensure email uniqueness.
AI-Generated Code from the Above Specification:
from flask import Flask, request, jsonify
from flask_sqlalchemy import SQLAlchemy
from werkzeug.security import generate_password_hash
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///users.db'
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(80), nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
password = db.Column(db.String(120), nullable=False)
@app.route('/users', methods=['POST'])
def create_user():
data = request.get_json()
hashed_password = generate_password_hash(data['password'])
new_user = User(name=data['name'], email=data['email'], password=hashed_password)
db.session.add(new_user)
db.session.commit()
return jsonify({'message': 'User created successfully'}), 201
@app.route('/users/<int:id>', methods=['PUT'])
def update_user(id):
user = User.query.get(id)
if not user:
return jsonify({'message': 'User not found'}), 404
data = request.get_json()
user.name = data.get('name', user.name)
user.email = data.get('email', user.email)
if 'password' in data:
user.password = generate_password_hash(data['password'])
db.session.commit()
return jsonify({'message': 'User updated successfully'})
if __name__ == '__main__':
db.create_all()
app.run(debug=True)
Crafting Human Intents : Development Alternatives to Prompts?
While this illustrates AI’s potential to handle complex specifications, it also highlights the limitations of this approach. As systems grow larger and more intricate, these specifications can become unwieldy and challenging to manage, which can hinder the scalability and maintainability of the AI-driven development process.
Achieving the goals of trust and system-level design will likely require more structured and formal methods of communication with the AI. Moving beyond simple prompts, the focus will shift to rich specifications that capture the nuances of system design. While UML is a widely used standard, it may not be the most suitable solution for AI-driven development. Several alternatives exist, each with its own strengths and weaknesses. For instance, domain-specific languages (DSLs) offer high expressiveness within a particular domain, while Business Process Model and Notation (BPMN) is useful for workflows and processes. Entity-relationship diagrams (ERDs) excel at data modeling, and state charts are ideal for capturing dynamic behavior. Formal specification languages provide rigor and precision, while ontologies capture rich semantic information.
The decision on which model or language to use—or whether to create something entirely new—will depend on factors like the complexity of the target systems, the desired level of abstraction, and the need for human interpretability. Ultimately, the most effective approach will likely involve a combination of these techniques, leveraging their strengths to create a robust and reliable bridge between human intent and AI-generated code. This is a topic that warrants further exploration and will be the focus of a
A question for you: As AI continues to advance, do you think it will ever fully understand human intentions and generate completely new systems without the need for code review? Should we pursue this level of AI capability? What would be the implications for developers and the industry?
Disclaimer! this article is polished via AI